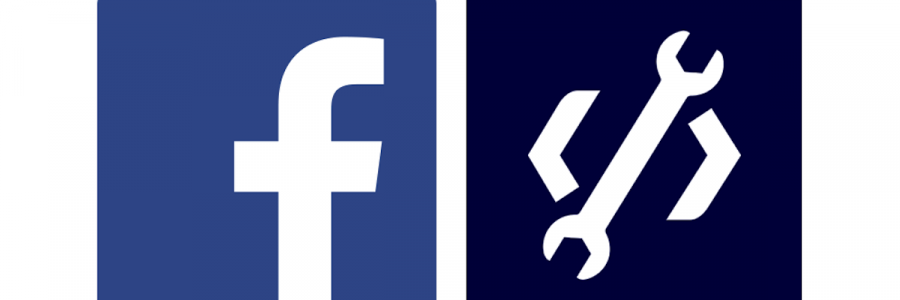
Get Business Manager data using Facebook Marketing API
So first thing I wanted to do when playing around with the Facebook Marketing API was to pull some data for the Business Managers (from here on in we’ll be calling them BMs). The format for an API call using the Facebook Marketing API (actually while we’re shortening things I’ll be calling this one FMAPI 🙂 ) using the SDK is Endpoint you want to connect to and fields you want plus any parameters passed in as arguements.
For the Business Endpoint we need to import the Business Class as follows:
from facebook_business.adobjects.business import Business
You can find all the available fields in the developer docs here however for now let’s just pull the name and created time. It’s alittle easier to declare the fields you want ahead of the call:
fields = ['name', 'created_time', ]
There are no parameters for this endpoint, so there’s no point trying to pass any in.
Before we make the call we need to initialise a connection to the FMAPI, to do this we need to import the Facebook Ads API class:
from facebook_business.api import FacebookAdsApi
When call init passing in our app id, app secret, access token and api version number:
FacebookAdsApi.init(app_id=app_id, app_secret=app_secret, access_token=access_token, api_version='v10.0')
This creates an FMAPI session we can use throughout to make API calls.
Now that we’ve initialised the FMAPI we can go ahead and call the BM endpoint passing in the id of the business manager we want to access (bm_id) and retrieve the data we need . Putting everything together your script should look like this:
from facebook_business.api import FacebookAdsApi from facebook_business.adobjects.business import Business FacebookAdsApi.init(app_id=app_id, app_secret=app_secret, access_token=access_token, api_version='v10.0') fields = ['name', 'created_time', ] business = Business(bm_id) business_info = business.api_get(fields=fields) print(business_info) # Or you ca just call it in one line business_info = Business(bm_id).api_get(fields=fields) print(business_info)
{
“created_time”: “2014-09-15T14:48:41+0000”,
“id”: “1234567890”,
“name”: “Business Manager Name”
}
In the next article we’ll hit some of the Edges of the Business Endpoint to get some more interesting data back, starting with AdAccounts, you can read it here.