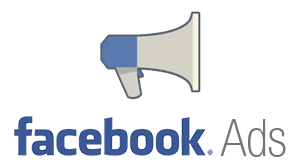
Get AdAccounts under Business Manager using Facebook Marketing API
In the lasts post we extracted data from the Business endpoint however it’s time to do a little more – let’s get the AdAccounts for a BM.
AdAccounts under a BM fall into one of two categories, either Owned Ad Accounts (created and owned by the BM) or Client AD Accounts (created by another BM and shared as an asset). We’ll need to make 2 API calls to get both the Client & Owned AdAccounts, we’ll then be able to combine the results.
First off you’ll need to initialise the Facebook API as outlined in the last post, we can then create a business object:
business = Business(bm_id)
Let’s get the names of the Owned AdAccounts:
fields = ['name'] business = Business(bm_id) ownedAdAccounts = business.get_owned_ad_accounts(fields=fields)
This’ll return a cursor object we can use to iterate through to get all the owned AdAccounts. We’ll first need to create a list to hold the accounts, to this we’ll append a dictionary of the AdAccount ID and AdAccount name. It’s worth noting the id is always returned throught the API so we don’t need to pas this in the fields.
allAdAccounts = list()
We can now iterate through the ownedAdAccounts and add the id and name.
allAdAccounts.append({'id': adAcc['id'], 'name': adAcc['name']}) for adAcc in ownedAdAccounts]
To get the client AdAccounts we call the get_owned_ad_accounts method on the business object:
clientAdAccounts = business.get_client_ad_accounts(fields=fields)
We can now add the clientAdAccounts to the list
allAdAccounts.append({'id': adAcc['id'], 'name': adAcc['name']}) for adAcc in clientAdAccounts]
allAdAccounts is a list of dictionaries contains all the AdAccounts visible in the Business Manager.
When getting the AdAccounts under a BM we’re using the AdAccounts edge – think of this as a join to AdAccounts, we can therefore use the fields of the AdAccounts endpoint to retrieve any additional fields.
Let’s get the account_status of the AdAccount, and filter out only the ‘ACTIVE’ AdAccounts.
allAdAccounts = list() fields = ['name', 'account_status'] business = Business(bm_id) ownedAdAccounts = business.get_owned_ad_accounts(fields=fields, params=params) [allAdAccounts.append({'id': adAcc['id'], 'name': adAcc['name'], 'account_status': adAcc['account_status']}) for adAcc in ownedAdAccounts if adAcc['account_status'] == 1]
We can filter out the client AdAccounts in the same way
clientAdAccounts = business.get_client_ad_accounts(fields=fields, params=params) [allAdAccounts.append({'id': adAcc['id'], 'name': adAcc['name'], 'account_status': adAcc['account_status']}) for adAcc in clientAdAccounts if adAcc['account_status'] == 1]
In the next post we’ll use the list of AdAccounts to go a layer deeper into Campaigns.